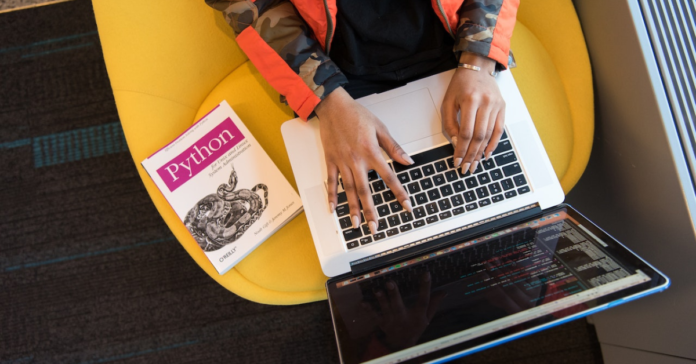
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones. In this blog post, we will discuss how to generate the Fibonacci series using the C programming language.
#include<stdio.h> #include<math.h> #include<stdlib.h> int main(void) { int n, curr=1, pre=0, temp; printf("Enter a number: "); scanf("%d", &n); printf("%d\t%d", pre,curr); // Displays previous and current numbers for(int i=3; i<=n; i++) { temp= curr; curr= curr + pre; pre= temp; printf("\t%d", curr); } return 0; }
First, we need to define some variables, namely curr and pre to store the current and previous numbers in the sequence, as well as a temporary variable temp to hold the current number while we update the values.
Next, we ask the user to enter a number to determine how many numbers in the series they want to display. We then print out the first two numbers in the sequence (0 and 1).
Finally, we use a for-loop to iterate through the remaining numbers in the sequence. In each iteration, we update the values of the current and previous numbers by adding the previous number to the current number and storing the result in the curr variable. We also use the temporary variable temp to hold the value of the current number while we update the values. We then print out the current number. When the loop completes, we return 0 to indicate that the program has executed successfully.
Overall, the code is a simple implementation of the Fibonacci series in C, demonstrating the use of variables, loops, and basic arithmetic operations.
Output
Enter a number: 5 0 1 1 2 3