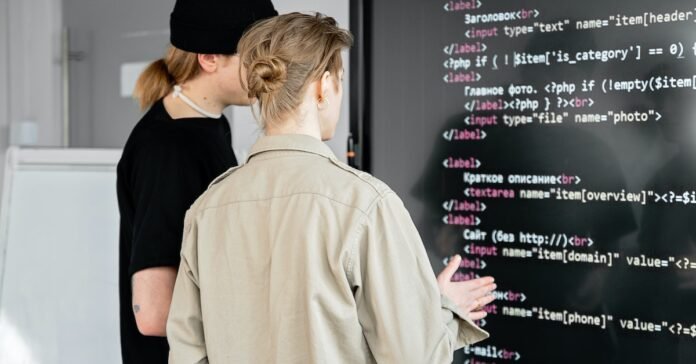
In this example, the user is asked to enter a number. The program will then calculate the factorial of the give number. For any given number the factorial is calculated as below,
Factorial of n (n!) = 1 * 2 * 3 * 4 * 5 * … n
Factorial of a negative number does not exist and the factorial of 0 is 1.
#include <stdio.h> int main() { int num, fact=1; printf("Please enter the number: "); scanf("%d", &num); if(num < 0) { printf("Factorial of a negative number does not exist.\n"); } else { for(int i=1; i<=num; i++) { fact = fact * i; } printf("Factorial = %d\n", fact); } return 0; }
First, two variables are declared, namely num and fact. fact is initialized to 1 beacuse later on we have to use fact for multiplication. If we do not initiliaze fact with 1 then it will use some garbage value for mulitiplication or if we initialize it to 0 then our result will always be 0. Hence, it is important to remember that fact=1. Next, the user is asked to enter a number which is stored in num.
if(num < 0) { printf("Factorial of a negative number does not exist.\n"); } else { for(int i=1; i<=num; i++) { fact = fact * i; } printf("Factorial = %d\n", fact); }
As mentioned before, the factorial of a negative number cannot be calculated. So, if user enters a negative number then following message is displayed on the console,
Factorial of a negative number does not exist.
If user enters a positive number then the program will go into the for-loop and will calculate the factorial. Finally, it displays the result on the console.
Output
Please enter the number: 6 Factorial = 720