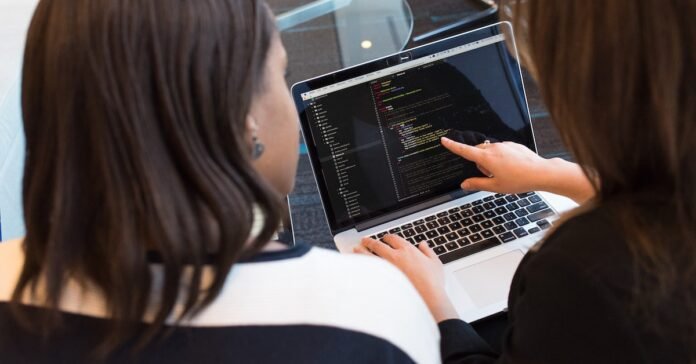
In this example, the user is asked to enter a string which will be then converted into uppercase.
#include <stdio.h> #include <string.h> int main() { char str[99]; printf("Please enter a string: "); fgets(str, sizeof(str), stdin); for(int i=0; str[i]!='\0'; i++) { if(str[i]>='a' && str[i]<='z') { str[i] = str[i]-32; } } printf("String in uppercase: %s", str); return 0; }
First, we have to include string.h library because we are working with strings. To store the entered string we are using an array str of type char. The array str has the capacity to store hundred elements in it. Next, we are using fgets() function to take the input from the user. To read strings with spaces in C, we can either use fgets() or gets(). fgets() is more safer than gets() function that’s why in this example we will rely on it.
for(int i=0; str[i]!='\0'; i++) { if(str[i]>='a' && str[i]<='z') { str[i] = str[i]-32; } } printf("String in uppercase: %s", str);
The for-loop will help us in traversing through the whole string until /0 is not found because every array ends with it. In the for-loop we have an if-condition. In if-condition if any character is found in lowercase it will convert it into uppercase by subtracting 32 from their ASCII value. Finally, the array str stores the result. The printf statment will then display the string on the console.
Output
Please enter a string: Hello World String in uppercase: HELLO WORLD